Introduction and Facebook App Setup
Hi folks,
I'm gonna explain that how you can implement facebook open authentication to your Application.
As we know Facebook is social giant and have lots and lots of data regarding users and they perform data analysis too on user activities to generate data. In real world all businessmen want their business grow rapidly and it can grow rapidly only if more and more people know about it, so they advertise by giving their business advertisement to websites who have more traffic and there is another way to reach people is through social sites like Facebook and their are several others too, but facebook is one of the sites that is accessed frequently by users.
I'm gonna explain that how you can implement facebook open authentication to your Application.
As we know Facebook is social giant and have lots and lots of data regarding users and they perform data analysis too on user activities to generate data. In real world all businessmen want their business grow rapidly and it can grow rapidly only if more and more people know about it, so they advertise by giving their business advertisement to websites who have more traffic and there is another way to reach people is through social sites like Facebook and their are several others too, but facebook is one of the sites that is accessed frequently by users.
Now coming to the point, Facebook launched its API's and tools on May 24, 2007 for third party application developers to access the Facebook data.
To access the Facebook data you must have a Facebook account first ( FYI :D ), after logging in to your account create your Facebook App from here: https://developers.facebook.com . Under the Menu Apps, select Add New App. see the below screen:
To access the Facebook data you must have a Facebook account first ( FYI :D ), after logging in to your account create your Facebook App from here: https://developers.facebook.com . Under the Menu Apps, select Add New App. see the below screen:
Now select the App Type you want to add according to your requirement, in my case i have a website so i will go for it.
In Next step give your App name and generate a unique App id for it.
Next step will ask you to select your App category, according my need i have to set a business App, you can select up to your requirement.
For a web application you can use localhost as a site url as in the below image, when you move it to production, you use the real one.Below is the screen where you can see the App Id and App Secret and some basic setting.
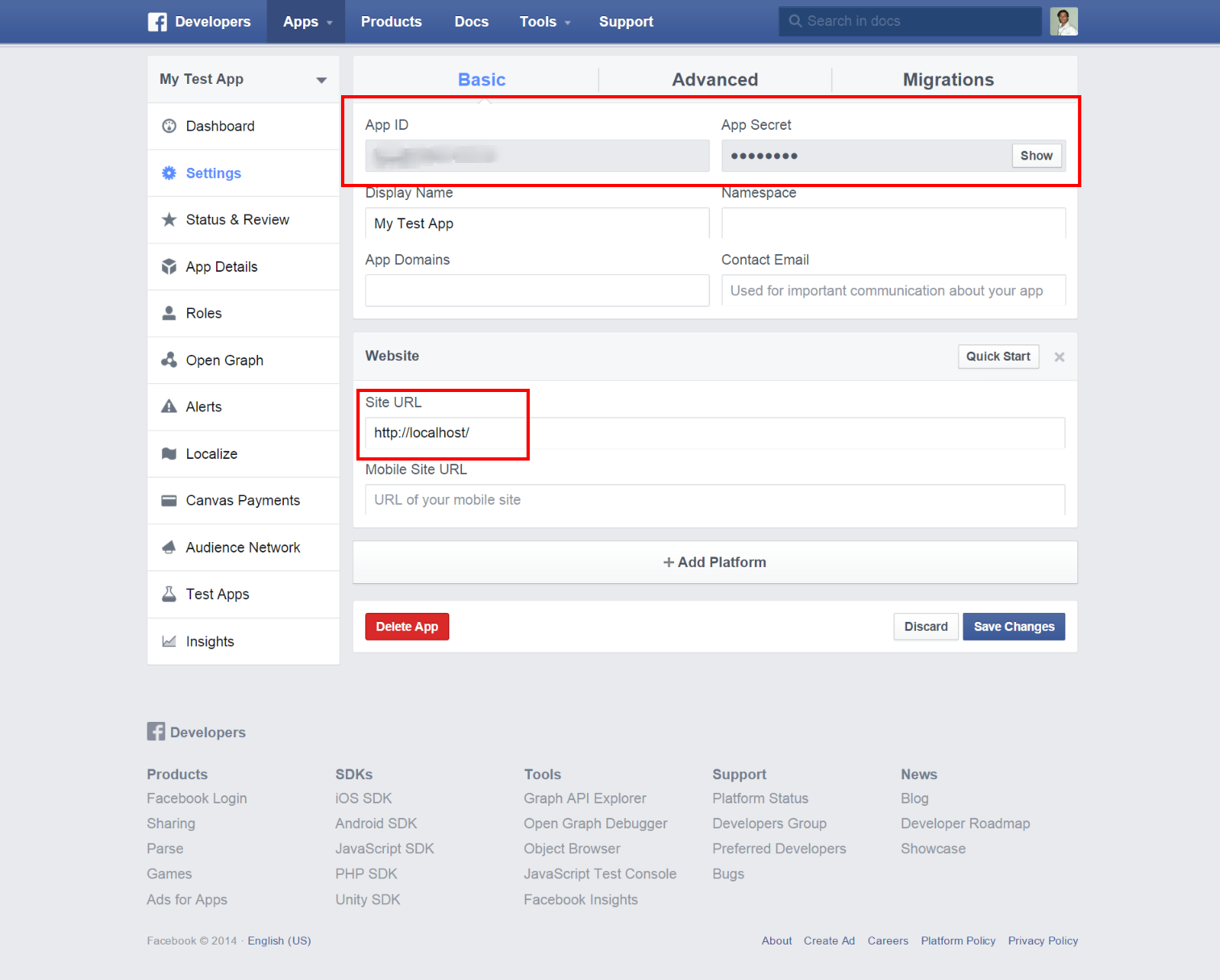
By default :
Your App has these permissions on, if you want more scopes (scopes are the set of permissions) to be added to your App then you have to click on START SUBMISSION button. After you complete all the settings of your App. Then its time move towards Code.
OAuth 2.0
Facebook implements Open Authentication system version 2.0 (OAuth 2.0). OAuth specifies a process for Facebook to authorize third-party access to their server resources without sharing their credentials. You can explore it more from here: http://oauth.net/2/Facebook provides Access Token through which your App can Access user's private data if user provides your App permission to access. Access Token has a short lifetime i.e it is valid for around 1 hour. Here's the facebook documentation over token's https://developers.facebook.com/docs/facebook-login/access-tokens
Facebook provides the access token's once, you have persist it somewhere in the management systems and when it expires you can ask for another access token by sending the previous expired token.
I will provide a code sample which do not uses facebook c# library for the OAuth process, this will clear the concept of Facebook OAuth process. Code is for Asp.net(web forms) using C# as script.
string OAuthURL = "https://www.facebook.com/dialog/oauth";
string OAuthTokenURL = "https://graph.facebook.com/oauth/access_token";
string client_id = "YOUR APP CLIENT ID;
string client_secret = "YOUR APP SECRET ID";
string scope = "email,user_birthday,user_location,user_about_me,user_likes";
string redirect_uri = "http://localhost:1234/facebook.aspx";
string serviceURL = "https://graph.facebook.com/me";
protected void Page_Load(object sender, EventArgs e)
{
OAuthLabel.Text = "OAuth Redirect Page";
if (Request["code"] != null)
{
//Build the form request from the parameters
string formData = "client_id=" + client_id +
"&client_secret=" + client_secret +
"&redirect_uri=" + redirect_uri +
"&grant_type=authorization_code" +
"&code=" + Request["code"];
//Exchange code for access token
System.Net.WebClient WC = new System.Net.WebClient();
WC.Headers.Add("Content-Type", "application/x-www-form-urlencoded");
var Results = WC.UploadString(new System.Uri(OAuthTokenURL), formData);
//Extract token from the results
string access_token = "";
try
{
JObject TokenData = JObject.Parse(Results);
access_token = TokenData["access_token"].ToString();
}
catch (Exception) //next try URL encoded data
{
string[] URLParts = Results.Split('&');
foreach (string S in URLParts) //extract the code from the URL
{
string[] param = S.Split('=');
if (param[0].Replace("?", "") == "access_token")
{
access_token = param[1];
//Build the form request from the parameters
string formData1 = "client_id=" + client_id +
"&client_secret=" + client_secret +
"&grant_type=fb_exchange_token" +
"&fb_exchange_token=" + access_token;
//Exchange code for long access token
System.Net.WebClient ExchangeWC1 = new System.Net.WebClient();
ExchangeWC1.Headers.Add("Content-Type", "application/x-www-form-urlencoded");
var Results1 = ExchangeWC1.UploadString(new System.Uri(OAuthTokenURL), formData1);
break;
}
}
}
//Call a service with the token
System.Net.WebClient ProfileWC = new System.Net.WebClient();
ProfileWC.Headers.Add("Authorization", "Bearer " + access_token);
Results = ProfileWC.DownloadString(new System.Uri(serviceURL));
//Display the users name..
JObject UserProfile = JObject.Parse(Results);
OAuthLabel.Text = "Hi, " + UserProfile["name"].ToString();
}
else //no "code" detected, redirect to OAuth service
{
string URL = OAuthURL + "" +
"?client_id=" + client_id +
"&scope=" + scope +
"&redirect_uri=" + redirect_uri +
"&response_type=code";
Response.Redirect(URL);
}
}
Facebook provides the access token's once, you have persist it somewhere in the management systems and when it expires you can ask for another access token by sending the previous expired token.
I will provide a code sample which do not uses facebook c# library for the OAuth process, this will clear the concept of Facebook OAuth process. Code is for Asp.net(web forms) using C# as script.
string OAuthURL = "https://www.facebook.com/dialog/oauth";
string OAuthTokenURL = "https://graph.facebook.com/oauth/access_token";
string client_id = "YOUR APP CLIENT ID;
string client_secret = "YOUR APP SECRET ID";
string scope = "email,user_birthday,user_location,user_about_me,user_likes";
string redirect_uri = "http://localhost:1234/facebook.aspx";
string serviceURL = "https://graph.facebook.com/me";
protected void Page_Load(object sender, EventArgs e)
{
OAuthLabel.Text = "OAuth Redirect Page";
if (Request["code"] != null)
{
//Build the form request from the parameters
string formData = "client_id=" + client_id +
"&client_secret=" + client_secret +
"&redirect_uri=" + redirect_uri +
"&grant_type=authorization_code" +
"&code=" + Request["code"];
//Exchange code for access token
System.Net.WebClient WC = new System.Net.WebClient();
WC.Headers.Add("Content-Type", "application/x-www-form-urlencoded");
var Results = WC.UploadString(new System.Uri(OAuthTokenURL), formData);
//Extract token from the results
string access_token = "";
try
{
JObject TokenData = JObject.Parse(Results);
access_token = TokenData["access_token"].ToString();
}
catch (Exception) //next try URL encoded data
{
string[] URLParts = Results.Split('&');
foreach (string S in URLParts) //extract the code from the URL
{
string[] param = S.Split('=');
if (param[0].Replace("?", "") == "access_token")
{
access_token = param[1];
//Build the form request from the parameters
string formData1 = "client_id=" + client_id +
"&client_secret=" + client_secret +
"&grant_type=fb_exchange_token" +
"&fb_exchange_token=" + access_token;
//Exchange code for long access token
System.Net.WebClient ExchangeWC1 = new System.Net.WebClient();
ExchangeWC1.Headers.Add("Content-Type", "application/x-www-form-urlencoded");
var Results1 = ExchangeWC1.UploadString(new System.Uri(OAuthTokenURL), formData1);
break;
}
}
}
//Call a service with the token
System.Net.WebClient ProfileWC = new System.Net.WebClient();
ProfileWC.Headers.Add("Authorization", "Bearer " + access_token);
Results = ProfileWC.DownloadString(new System.Uri(serviceURL));
//Display the users name..
JObject UserProfile = JObject.Parse(Results);
OAuthLabel.Text = "Hi, " + UserProfile["name"].ToString();
}
else //no "code" detected, redirect to OAuth service
{
string URL = OAuthURL + "" +
"?client_id=" + client_id +
"&scope=" + scope +
"&redirect_uri=" + redirect_uri +
"&response_type=code";
Response.Redirect(URL);
}
}